Selenium is an open source Salesforce test automation tool used for browser based ‘Regression Testing‘. It is used to automate Cross-Browser testing across different platforms, and supported by multiple programming languages.
Go to the following link to configure selenium webdriver on your machine
http://www.codeproject.com/Tips/826914/How-to-Setup-and-Configure-Selenium-Webdriver-With.
Here, we are going to test a Salesforce custom application (i.e. Leave Management) using the Selenium web driver. The Script is written in Java.
Let’s discuss the Salesforce application and its business functionality we are going to test. Here is the scenario: an Organization uses Salesforce application for leave management process. ‘Leave Management’ is a custom application used to apply for leave from the employees’ Salesforce account. Once an employee has applied leave from their account, it’s automatically submitted to their reporting managers for approval. The Manager would either ‘Approve’ or ‘Reject’ the leave request from their account based on the number of days leave requested.
In Selenium, we are using a JXL jar to get input data from an Excel file and write the output into an Excel file.
Input File:
JXL jar is used to read the below input data from the Excel file.

Figure – 1
Read Excel data using JXL:
The below code is used to read the excel data using the JXL jar file. This code reads the username and password from the file. You can read rest of the data as same as we have explained for username and password columns.
//Read excel data FileInputStream fi = new FileInputStream ("//home//mstemp122//Auto_Input.xls"); //Open Workbook Workbook w = Workbook.getWorkbook(fi); //Open sheet Sheet s = w.getSheet(0); for(int row =1; row < s.getRows(); row = row+1 ) { int aa = s.getRows(); System.out.println("Row Limit :"+aa); //Log in as an employee driver.get ("https://login.salesforce.com"); driver.manage().window().maximize(); //Read username from excel file String username = s.getCell(1, row).getContents(); System.out.println("username :"+username); driver.findElement(By.xpath(".//*[@id='username']")).sendKeys(username); Thread.sleep(5000); //Read password from the Excel file String password = s.getCell(2, row).getContents(); System.out.println("Password: "+password); driver.findElement(By.xpath(".//*[@id='password']")).sendKeys(password); Thread.sleep(5000); driver.findElement(By.xpath(".//*[@id='Login']")).click(); driver.manage().timeouts(). implicitlyWait(90, TimeUnit.SECONDS); String e = driver.findElement(By.id("tsidButton")).getText(); Thread.sleep(1000); System.out.println("Current Application: " +e); }
An employee wants to apply leave for three days. This request needs to get approved by their reporting managers. The company rule is that if Leave is applied for less than or equal to two days, it should go to ‘Project Manager’ alone, if it is more than two days, it should go to both ‘Project Manager’ and ‘HR’ (See the image below).
Figure – 2
Now, the employee is going to apply for leave through the “Leave Management” application.
Following are the 3 testing scenarios that we covered in the Selenium automation,
Scenario 1:
Create a new “Leave Application” record with invalid Employee ID. It should throw an error message as mentioned in the below screenshot, and the Leave record shouldn’t be saved.
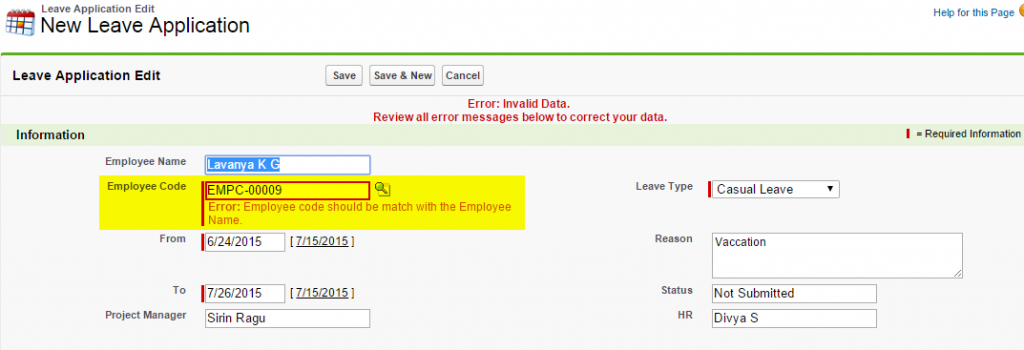
Figure – 3
Scenario 2:
Create a new “Leave Application” record with valid input. It should be saved and the record should be submitted for the approval.
Once the record is submitted for approval, the approver would receive an email regarding the leave submission.
- Login as a Project Manager and approve the leave request.
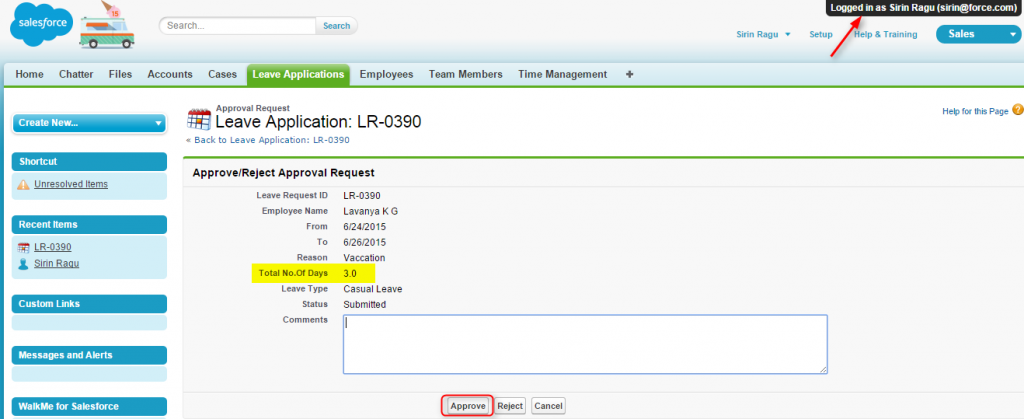
Figure – 4
- Login as a HR Staff and approve the leave request
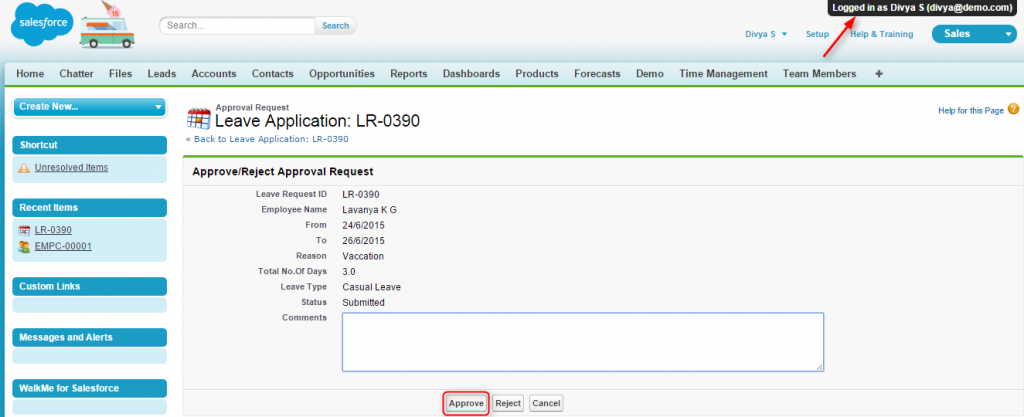
Figure – 5
- Finally, the leave request is approved.
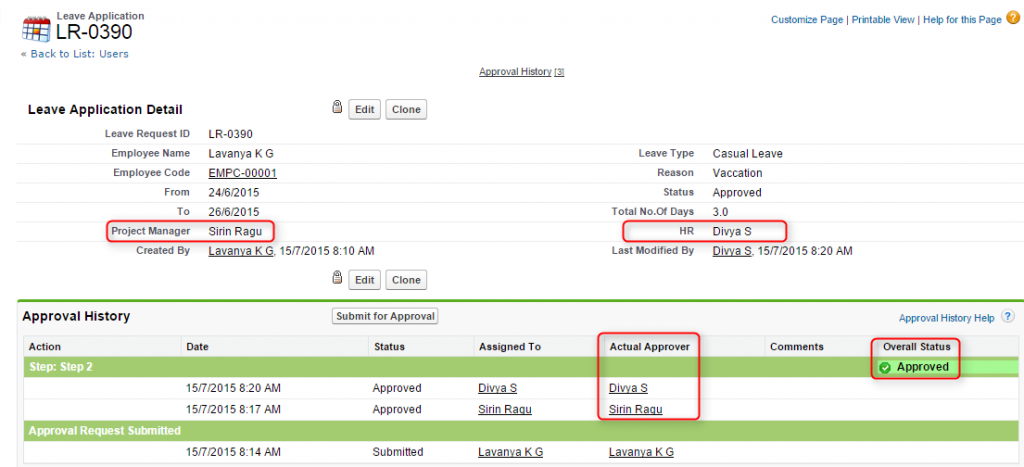
Figure – 6
Scenario 3:
Employee shouldn’t be able to apply leave for the dates already applied. If they try to apply leave for the same dates again, it should throw an error message as shown in the below screenshot.
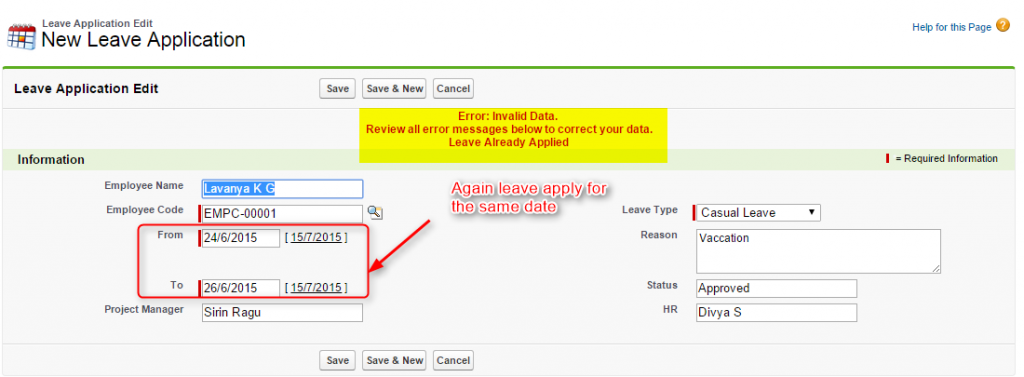
Figure – 7
Output File:
Once the automation is complete, it automatically generates the below output file with Scenario, Expected Result, Actual Result and Result columns.
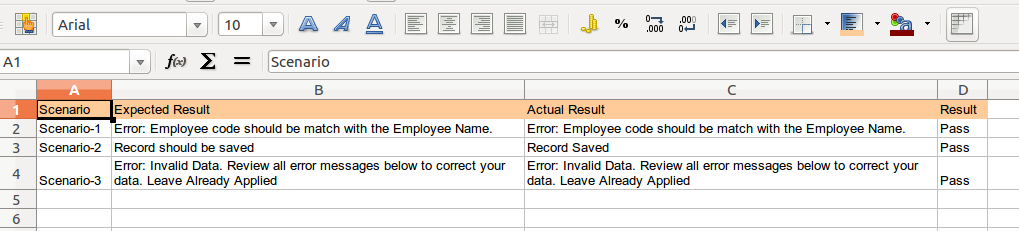
Figure – 8
Code for generating the output file using JXL:
The below code is used to write the test results into the output file.
//Start to write output data into excel //Create an excel file for output FileOutputStream resultfi = new FileOutputStream ("//home//mstemp122//Auto_Output_File.xls"); //Create an instance for the above file WritableWorkbook xlfile = Workbook.createWorkbook(resultfi); //Create a sheet WritableSheet xlsheet = xlfile.createSheet("Output", 0); //Create data into the above created sheet Label Colhead1 = new Label(0,0, "Scenario"); Label Colhead2 = new Label(1,0, "Expected Result"); Label Colhead3 = new Label(2,0, "Actual Result"); Label Colhead4 = new Label(3,0, "Result"); //Label Sce2Row1 = new Label(2,1, sce1error1); Label Sce1Row1 = new Label(0,1, "Scenario-1"); Label Sce1Row2 = new Label(0,2, "Scenario-2"); Label Sce1Row3 = new Label(0,3, "Scenario-3"); Label ExpRow1 = new Label(1,1, "Error: Employee code should be match with the Employee Name." ); if(err1.equals(sce1error1)) { Label ActRow1 = new Label(2,1, sce1error1 ); xlsheet.addCell(ActRow1); Label Result1 = new Label(3,1, "Pass"); xlsheet.addCell(Result1); } else { Label ActRow1 = new Label(2,1, "NA"); xlsheet.addCell(ActRow1); Label Result1 = new Label(3,1, "Fail"); xlsheet.addCell(Result1); } Label ExpRow2 = new Label(1,2, "Record should be saved"); if(autonum != null) { Label ActRow2 = new Label(2,2, "Record Saved"); xlsheet.addCell(ActRow2); Label Result1 = new Label(3,2, "Pass"); xlsheet.addCell(Result1); } else { Label ActRow2 = new Label(2,2, "Record not saved"); xlsheet.addCell(ActRow2); Label Result1 = new Label(3,2, "Fail"); xlsheet.addCell(Result1); } Label ExpRow3 = new Label(1,3, "Error: Invalid Data. Review all error messages below to correct your data. Leave Already Applied"); if(err2.equals(err3)) { Label ActRow3 = new Label(2,3, err3); xlsheet.addCell(ActRow3); Label Result3 = new Label(3,3, "Pass"); xlsheet.addCell(Result3); } else { Label print1 = new Label(2,3, "Verify the data"); xlsheet.addCell(print1); Label Result3 = new Label(3,3, "Fail"); xlsheet.addCell(Result3); } //Adding cell values xlsheet.addCell(Colhead1); xlsheet.addCell(Colhead2); xlsheet.addCell(Colhead3); xlsheet.addCell(Colhead4); xlsheet.addCell(Sce1Row1); xlsheet.addCell(Sce1Row2); xlsheet.addCell(Sce1Row3); xlsheet.addCell(ExpRow1); xlsheet.addCell(ExpRow2); xlsheet.addCell(ExpRow3); //Writing the values into excel xlfile.write(); xlfile.close(); //To stop the loop row = aa + 1;