Lightning Component, a single-page application, comprises of dynamic and responsive user interfaces which use JavaScript on the client side and Apex on the server side. Sometimes it is required to create the lightning component at runtime and destroy them. In order to instantiate any lightning component dynamically in the client-side JavaScript code, use $A.createComponent() and to create multiple components, use $A.createComponents(). Creating and destroying the components in the runtime helps free up the memory used by the browser and makes the Lightning application to run fast.
Syntax:
$A.createComponent(String type, Object attributes, function callback)
- type (String) : Type of component to create , ex: lightning:button.
- Attributes : Map of all parameters required for new component, including the local Id (aura:id).
- Callback (cmp, status, errorMessage): Callback is used to invoke after a component is created. It has three parameters. It can be used to append newly created component to target component and we can place the new component inside div tags to display.
- Cmp – New component is created, and it enables to do some stuff with the newly created component. if there is an error, cmp will be null.
- Status – Possible status of the call values are SUCCESS, INCOMPLETE, or ERROR.
- errorMessage – When the status is ERROR.
Following is the syntax of an example that narrates how to create a lightning component which will have lightning buttons and destroy them dynamically.
CreateAndDestroyComp.cmp:
<aura:component >
<lightning:button label=”Create Button” onclick=”{!c.createButtonDynamically}” variant=”brand”/>
<lightning:button label=”Destroy All buttons” onclick=”{!c.removeButtonDynamically}” variant=”destructive”/>
<br/>
<div style=”margin:auto; height:600px; width:400px; border:2px ridge red;” aura:id=”newtag”>
<p>Dynamically creating and Destroying Lightning Component</p>
{!v.body}
</div>
</aura:component>
In the above client-side controller for CreateAndDestroyComp.cmp, it calls $A.createComponent() to create a ui:button with a local ID and a handler for the press event. The function(dynamicButton, status, errorMessage) callback appends the button to the body of the C:createAndDestroyComp. The new button which is created dynamically is passed as the first argument to the callback.
CreateAndDestroyCompController.js:
({
createButtonDynamically : function(component, event, helper) {
//Creating button dynamically
var db=component.find(“newtag”);
$A.createComponent (
“ui:button”,
{
“label”:”New Button”+db.get(“v.body”).length,
“press”: component.getReference(“c.showPressedButtonLabel”)
},
function(dynamicButton, status, errorMessage)
{
//Add the new button to the body array
if (status === “SUCCESS”) {
var bdy=db.get(“v.body”);
bdy.push(dynamicButton);
db.set(“v.body”,bdy);
}
else if (status === “INCOMPLETE”) {
console.log(“No response from the server!”)
}
else if (status === “ERROR”) {
console.log(“Error: ” +errorMessage);
}
}
);
},
removeButtonDynamically : function(component, event, helper){
component.find(“newtag”).set(“v.body”,[]);
//Destroying the component dynamically.
},
showPressedButtonLabel : function(component, event, helper){
alert(‘You pressed:’+event.getSource().get(“v.label”));
}
})
CreateAndDestroy.app:
<aura:application extends=”force:slds” >
<c:createAndDestroyComp />
</aura:application>
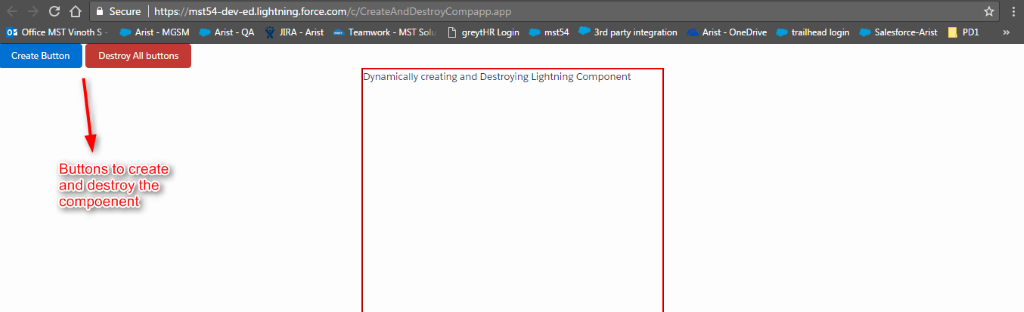
Whenever a user clicks the Create Button, the controller will create a new button dynamically and append it in <div/> tag. The Controller always checks for v.body which returns the body of component and will check the length of body and append label to new button.
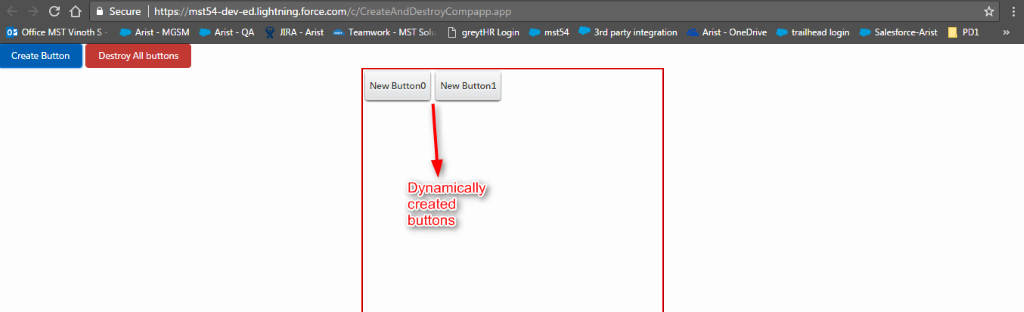
Destroying Dynamically created components:
When the dynamically created component declared in the markup is no longer in use, the framework automatically destroys it and frees up its memory to run the application faster. In the above controller to destroy the component i.e. to delete all buttons present in div tag, we find the div tag with aura:id and set the body as blank array. When the user clicks Destroy All Buttons button, then all buttons created dynamically will be destroyed dynamically.
component.find(“newTag”).set(“v.body”,[]);
In order to remove all the content of any components, simply set the v.body to blank array as shown in the below,
Example:
var bdy = component.get(“v.body”);
bdy .set(“v.body”,[]);
If you create a component dynamically in JavaScript and that component isn’t added to a facet (v.body or another attribute of type Aura.Component[]), you have to destroy it manually using Component.destroy() to avoid memory leaks.
Dependencies while creating custom component dynamically:
When we creat a custom component like c: createAndDestroyComp and when we want to create it dynamically and to use it in another component c:listComponent, then we have to specify the c: createAndDestroyComp component as a dependent component inside c:listComponent. The framework will automatically track the dependencies between definitions such as components defined in the markup. However, some dependencies aren’t easily discoverable by the framework for example, if you dynamically create a component that isn’t directly referenced in the components markup, then use <aura:dependency > to ensure that the component and its dependencies are sent to client when it’s needed.
<aura:component>
<aura:dependency resource=”markup://c:myCustomDialog”/>
</aura:component>
Considerations:
- The createComponent() method supports both client-side and server-side component creation.
- No server call is made when there is no server dependencies and the definition already exists on the client using declared dependencies.
- When no server-side dependencies are found, createComponent() method will execute in client-side.
- No limit in component creation on the client side.
- Can create up to 10,000 components in one server request.
References: