Salesforce Errors while developing applications–which involve apex Classes, Triggers, Visualforce pages, etc.—in Salesforce, we may come across different kinds of common errors. These error codes are enumerated messages that correspond to faults in a specific piece of code in application. Writing programs that work when everything goes expected is a good start; however, building applications/programs that behave properly when encountering unexpected conditions is where it really gets challenging. (Marijn Haverbeke, 2013). This article explains some of the common errors in Salesforce and the ways to resolve them in an effective manner.
1.Mixed DML Operation Error:
Reason: When you try to combine DML operations for both Setup and Non-Setup objects in a same transaction (Where Setup objects such as Group, Group member, Queue SObject, User. Non-Setup Objects such as Standard and Custom Objects).
Resolution: We can use @future annotation to do DML operation. It will split the transactions.
Another approach is to use same logic in a batch class to overcome.
2.Cpu time limit exceed:
Reason 1: Based on CPU usage, Salesforce has a timeout limit for each transaction. Due to consumption of too much CPU time limit in a transaction, this error could occur.
Reason 2: If you use nested for-loop (for-loop inside another for-loop), then you must use Map(s) to resolve the issue, as it is one of the common reasons for CPU Time Limit
For { for { } }
Counted:
- All Apex Code.
- Workflow execution.
- Library functions exposed in Apex.
Not Counted:
- Database Operations (DML)
- SOSL
- SOQL
- HTTP Callouts.
3.System.QueryException: Non-selective query against large object type:
Reason: If you query records on object that returns more than 200,000 records and without query filters, it’s possible that you’ll receive the error, “System.QueryException: Non-selective query against large object type.” We’ll go over the a few possible fixes.
Resolution: It’s best to try to have that field indexed by checking the “external ID” checkbox when you create that field. Also, as a best practice, always use WHERE clause in your SOQL statements to read the records.
Example: [Select id, Name, Type from Account WHERE Type = “Prospect”];
Finally, filter out all null records in your Apex query.
4.Too many SOQL Queries:
Reason: We can run up to a total of 100 SOQL queries in a single transaction or context. All the SOQL queries in triggers fired from one call or context will be counted against the limit of 100. When the number of SOQL queries exceed the limit in a single transaction, then this error is thrown by Salesforce.
Resolution: To fix this issue, you’ll need to change your code in such a way that the number of SOQL fired is less than 100. If you need to change the context, then you can use @future annotation which will run the code asynchronously. Here are some best practices that will stop the error messages.
Governor Limit:
1. Since Apex runs on a multi-tenant platform, the Apex runtime engine strictly enforces limits to ensure code doesn’t monopolize shared resources. Learn about the Governor Limits
2. Avoid SOQL queries that are inside for loops.
3. Check out the Salesforce Developer Blog where you can find Best Practices for Triggers.
4. Review best practices for Trigger and Bulk requests on our Force.com Apex Code Developer’s Guide.
5. Be sure you’re following the key coding principals for Apex Code in our Developer’s Guide.
Important: Salesforce cannot disable the Governors Limit or raise it. Following the best practices above should ensure that you don’t hit this limit in the future.
5.Non-recursive Parameterized interface method generating infinite recursion error: “Maximum stack depth reached: 1001”:
Reason 1: Let’s say in ‘after update’ trigger, a Developer is performing update operation and this leads to recursive call.
Resolution: We can create a class with a static Boolean variable with default value true. In the trigger, before executing your code keep a check that the variable is true or not. After you check, make the variable as false.
Example Code:
Class: public class ContactTriggerHandler { public static Boolean isFirstTime = true; } Trigger: trigger ContactTriggers on Contact (after update) { Set<String> accIdSet = new Set<String>(); if(ContactTriggerHandler.isFirstTime) { ContactTriggerHandler.isFirstTime = false; for(Contact conObj : Trigger.New) { if(conObj.name != 'Test') { accIdSet.add(conObj.accountId); } } // any code here } }
6. Too many Query rows:
Reason: The exception is being thrown because the maximum number of rows we can return in SOQL calls in Apex is 50000.
Resolution: Limit the query results by adding limit. If you want to insert additionally, you will need to use Batch Apex in which the 50K limit counts per batch execution. This limit Counts for each transaction and these limits are reset for each execution of a batch of records in the execute method.
7. Error: System.ListException: List index out of bounds: 0
Reason: Our Query has returned no records that match the criteria. If you then attempt to access an element at row 0, this will throw an error as that row doesn’t exist.
Resolution: Initially Always Check whether the list has records or not.
Error Code:
List<custom obj__c> lrecords=[select Id,name from custom obj__c ]; lrecords[0].Fileld1__c=2; lrecords[0].Fileld2__c=3; update lrecords; Modified Code: List<custom obj__c> lrecords=[select Id,name from custom obj__c ]; If(lrecords.size()>0) { lrecords [0].Fileld1__c=2; lrecords[0].Fileld2__c=3; update lrecords; }
8. Error: Sobject row was retrieved via SOQL without querying the requested field:
Reason: This Error is caused by using the field that is not queried in the SOQL query.
Wrong Code:
Code: Id ids = ‘0012800001EOUIl’; List<Contact> c =new list<Contact>([Select id from Contact WHERE id =” ids]); String Conname = c[0].Name; System.Debug(‘@@@@’+c[0].id); Resolution: We must add the field name in the query. Modified Code: Id ids = ‘0012800001EOUIl’; List<Contact> c =new list<Contact>([Select id,name from Contact WHERE id =” ids]); String Conname = c[0].Name; System.Debug(‘@@@@’+c[0].id);
9. System.QueryException: List has no rows for assignment to SObject:
Reason: This error is caused when the query cannot get the results back.
Resolution:
Id ids = null’; Contact c = [Select id,name from Contact WHERE id =” ids]; System.Debug(‘@@@@’+c.id); Modified Code: Id ids = ‘Null’; If(ids!=Null) { Contact c = [Select id,name from Contact WHERE id =” ids]; System.Debug(‘@@@@’+c.id); }
10. System.AsyncException: Rate Limiting Exception: AsyncApexExecutions Limit exceeded:
Reason: This error occurs when we are hitting an org-wide 24 hour rolling limit on asynchronous Apex.
The maximum number of asynchronous Apex method executions (batch Apex, future methods, Queueable Apex, and scheduled Apex) per a 24-hour period: 250,000 or the number of user licenses in your organization multiplied by 200– whichever is greater”.
11. System.AsyncException: Maximum callout depth has been reached:
Reason: Queueable Apex Limits
The execution of a queued job counts against the shared limit for asynchronous Apex method executions.
Resolution:
- You can add up to 50 jobs to the queue with System.enqueueJob in a single transaction.
- No limit is enforced on the depth of chained jobs, which means that you can chain one job to another job and repeat this process with each new child job to link it to a new child job. For Developer Edition and Trial organizations, the maximum stack depth for chained jobs is 5, which means that you can chain jobs four times and the maximum number of jobs in the chain is 5, including the initial parent queueable job.
- When chaining jobs, you can add only one job from an executing job with System.enqueueJob, which means that only one child job can exist for each parent queueable job. Starting multiple child jobs from the same queueable job isn’t supported.
12.System.DmlException: Insert failed. First exception on row2; firsterrorINSUFFICIENT_ACCESS_ON_CROSS_REFERENCE_ENTITY, insufficient access rights on cross-reference id: []:
Reason: This error normally happens when a DML operation is performed with a wrong record Id.
13.System.NullPointer Exception: Attempt to Dereference a null object:
Reason: If we use the collections without initializing and if we use any methods of a null object or refer a null variable, null pointer or object exception will occur.
Sample Code:
List<Contact> contactList; Contact contactIns = new Contact(); ContactIns.LastName = 'test'; ContactList.add(contactIns );
14.Error: Invalid Data.: Apex trigger Acc caused an unexpected exception, contact your administrator: Acc: execution of AfterInsert caused by: System.DmlException: Insert failed. First exception on row 0 with id 0032800000vimdqAAA; first
error: INVALID_FIELD_FOR_INSERT_UPDATE, cannot specify Id in an insert call: [Id]: Trigger.Acc: line 11, column 1:
Reason: While inserting a record in Trigger. the dml should be outside the for loop.
Sample Code:
This is the wrong code:
trigger Acc on Account (after insert) { List<Contact> ListCont =New List<Contact>(); for(Account acnt : Trigger.New){ for(integer i=0;i<3;i++){ Contact Cont=new Contact(); cont.Lastname=acnt.name+'ContApex1'; cont.accountid=acnt.id; ListCont.add(cont); insert ListCont; } } }
15.Error: Record is Read only:
Reason: When you are doing an After trigger, Trigger.new is read only. If you want to make changes to it, then it is best to do it in a Before trigger.
Also, your trigger is not bulkified. If more than one record is updated at a time, then only the first one will be evaluated.
Wrong Code:
trigger sample on Account (after insert) { for (Account sample: Trigger. New) { sample. Email =sample.Email__c; } }
Modified Code:
trigger sample on Account (after insert) { List<Account> Acclis = [SELECT Id,Name,Email,Email__C FROM Account WHERE Id IN: Trigger.newMap.keySet()]; for (Account Acc: Acclist){ Acc.Email = Acc.Email__c; }Update Acclist;}
16.Required Field Missing:
Reason: In DML operation, we have missed the required field for the sObject.
Code: trigger Acc on Account (after insert) { List<Contact> ListCont =New List<Contact>(); for(Account acnt : Trigger.New) { for(integer i=0;i<3;i++) { Contact Cont=new Contact(); cont.accountid=acnt.id; ListCont.add(cont); } } insert ListCont; }
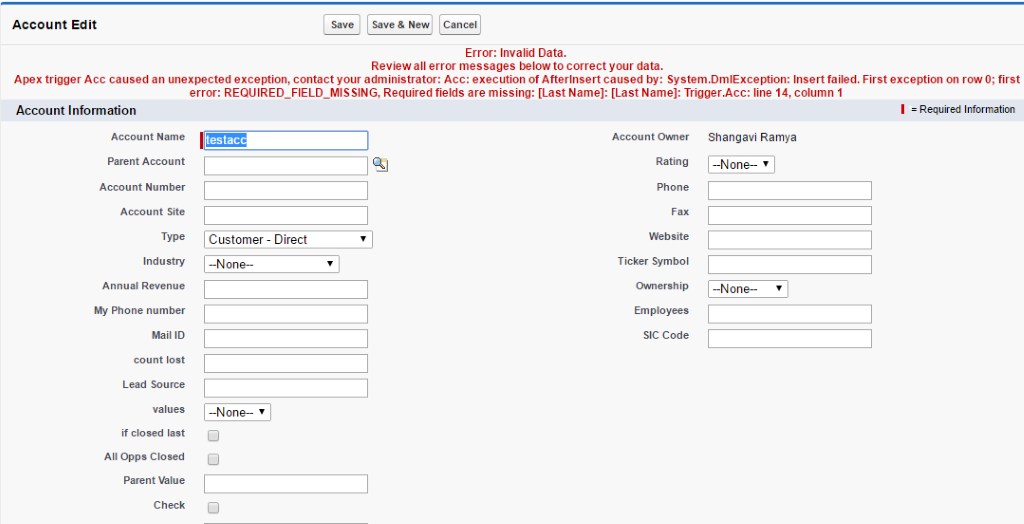
Modified Code:
trigger Acc on Account (after insert) { List<Contact> ListCont =New List<Contact>(); for(Account acnt : Trigger.New) { for(integer i=0;i<3;i++) { Contact Cont=new Contact(); Cont.Lastname = acnt.Name+’Sample’; //This is the required field in Contact cont.accountid=acnt.id; ListCont.add(cont); } } insert ListCont; }
17. Error: Compile Error: Invalid foreign key relationship: Account.Site at line 15 column 12:
Reason: While assigning a field value, the Syntax should be SobjectName.FieldName or SObjectName.method()
Sample Code (Error) trigger sample on Account(before insert, before update){ integer i = 0; Map<String, Account> accountMap = new Map<String, Account>{}; for (Account acct : System.Trigger.new) accountMap.put(acct.Name,acct); for (Account oldAccount: [SELECT Id, Name,Site FROM Account WHERE Name IN:accountMap.KeySet()]){ Account newAccount = accountMap.get(oldAccount.Name); i = newAccount.Site.length; } } Modified Code: trigger sample on Account (before insert, before update){ integer i = 0; Map<String, Account> accountMap = new Map<String, Account>{}; for (Account acct : System.Trigger.new) accountMap.put(acct.Name,acct); for (Account oldAccount : [SELECT Id,Name,Site FROM Account WHERE NameIN :accountMap.KeySet()]) { Account newAccount = accountMap.get(oldAccount.Name); i = newAccount.Site.length(); }}
18.System.security. NoAccessException: Update access denied for RecordType controller action methods may not execute:
Reason: This error means that the profile that executes the apex code has no permission to create the sObject.
Resolution: Check whether do you have access to the apex code, or to modify your profile
– change the first line of apex code from “public with sharing…” to “public without sharing…”.
or
- Add create permission to your profile (setup / manage users / profiles / your profile / check create checkbox in account object)
19. System.SObjectException: DML statement cannot operate on trigger. New or trigger. Old: Trigger.extractnumericvalues:
Reason: We cannot perform DML operation in before insert/before update in same record. Also, refer the Order of Execution.
Sample Code:
trigger extractnumericvalues on Account (before insert) { list<account> acList = new list<account>(); Matcher matcher; Pattern pat; for(account acc : trigger. New) { if(acc.AccountNumber != null) { pat = Pattern.compile('[0-9]'); matcher = pat. matcher(acc. Account Number); Boolean matches = matcher.find(); acc.Copy_Me__c = matcher.group(); acList.add(acc); } } insert acList;// Error will flow here because we cannot do dml for the same record. }
20.Error: Invalid Data Review all error messages below to correct your data. Apex trigger trigger task caused an unexpected exception, contact your administrator: trigger task: data changed by trigger for field Pick Val: bad value for restricted picklist field: Val10:
Reason: In trigger, when we try to insert new values into the picklist rather than using the existing values in the picklist, this error will fire during insertion.
Resolution:
Solution 1: To accept the new values apart from the existing picklist values: Goto View Fields–>Corresponding Picklist–>Edit–>Picklist Options–>uncheck the “Restrict picklist to the values defined in the value set”.
Solution 2: Adding a necessary picklist value to the existing picklist.
References