Now a day, the businesses constantly look for modern trend/environment to collaborate with clients and have the need to integrate their existing enterprise systems with the Cloud based service such as Salesforce platform. Let us see how to integrate the Salesforce with Microsoft .Net applications using SOAP API?
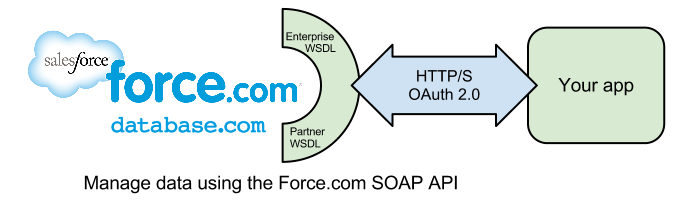
This is a common integration method required to be executed on any Microsoft .NET supported platform. The following steps are involved to establish the connection with Console application.
• Generate API WSDL in Salesforce
• Add a Web service reference to the .Net application
• Establish API reference within Salesforce
Generating API WSDL in Salesforce:Â
Step 1: WSDL files are retrieved via the standard user interface in Salesforce organization by navigating to Setup > Develop > API. Choose the appropriate Partner WSDL.
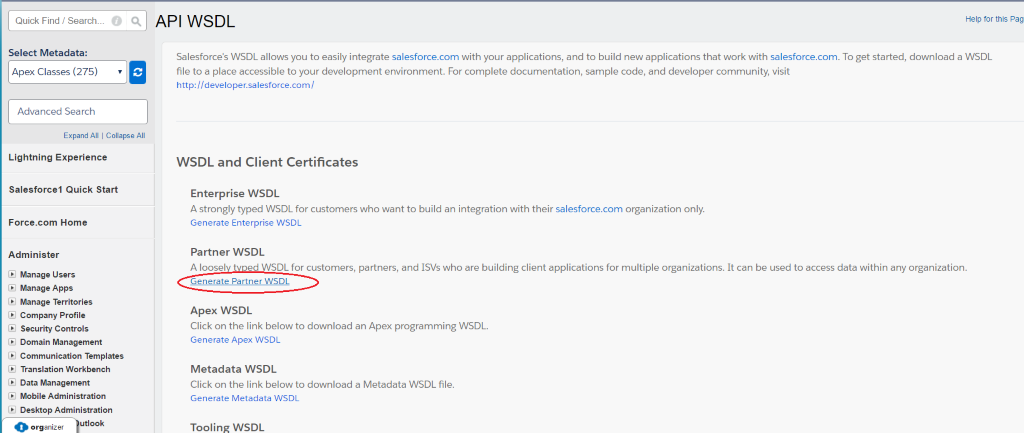
Step 2: Click the Generate button.
After that, you will receive the WSDL file in XML format. Store the below generated file in your local disk.
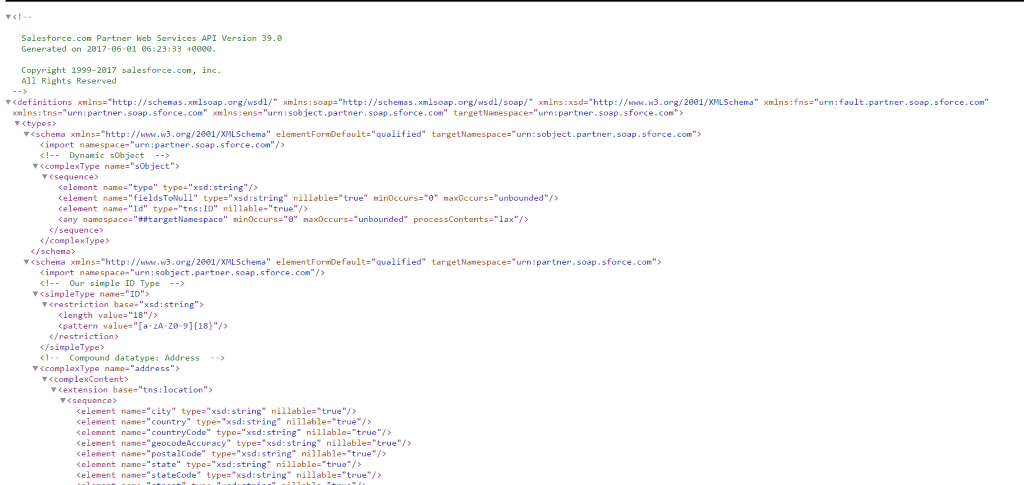
Add Web service reference to the .Net application:
Step 3: Reference the generated file in the Visual studio console application.
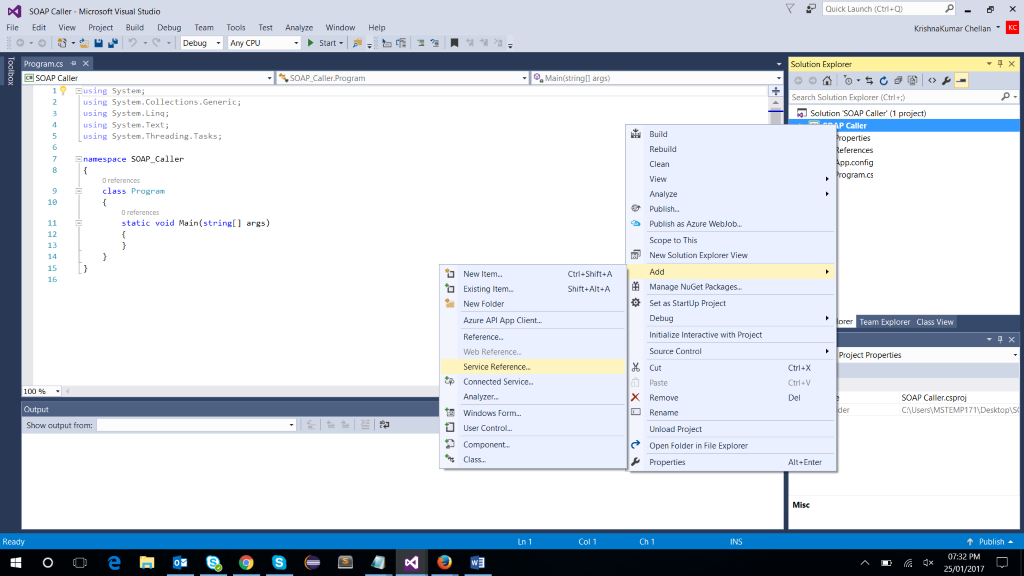
Step 4: Add the endpoint URL in the SOAP API Service Reference.
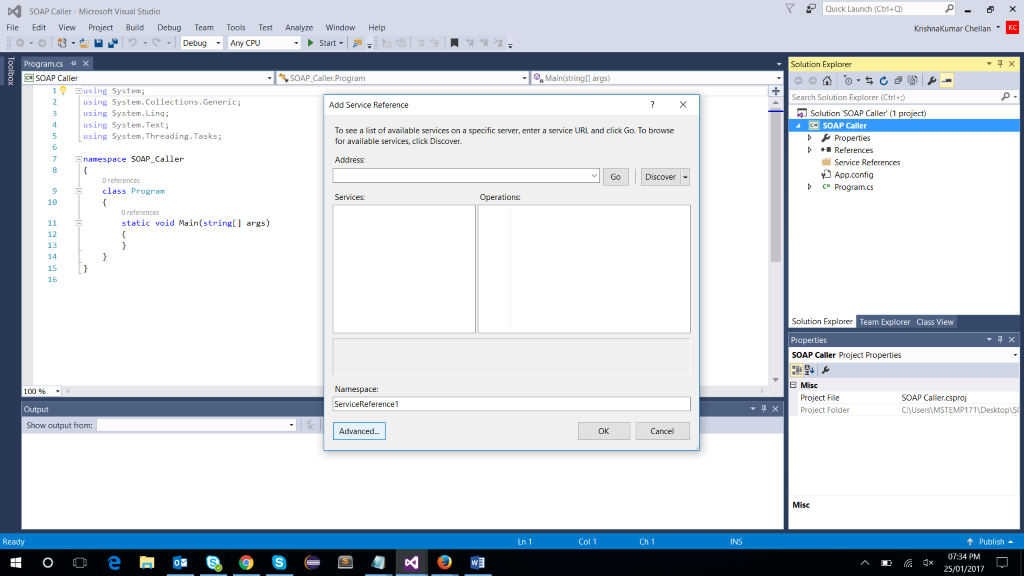
Step 5: Click the Add Web Reference button.
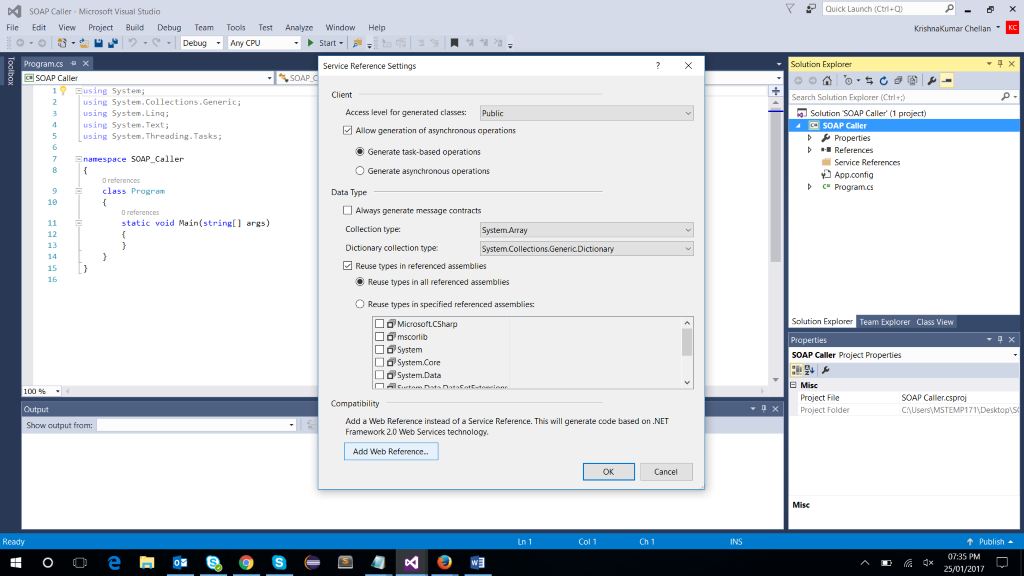
Step 6: Enter the Salesforce user credential details.
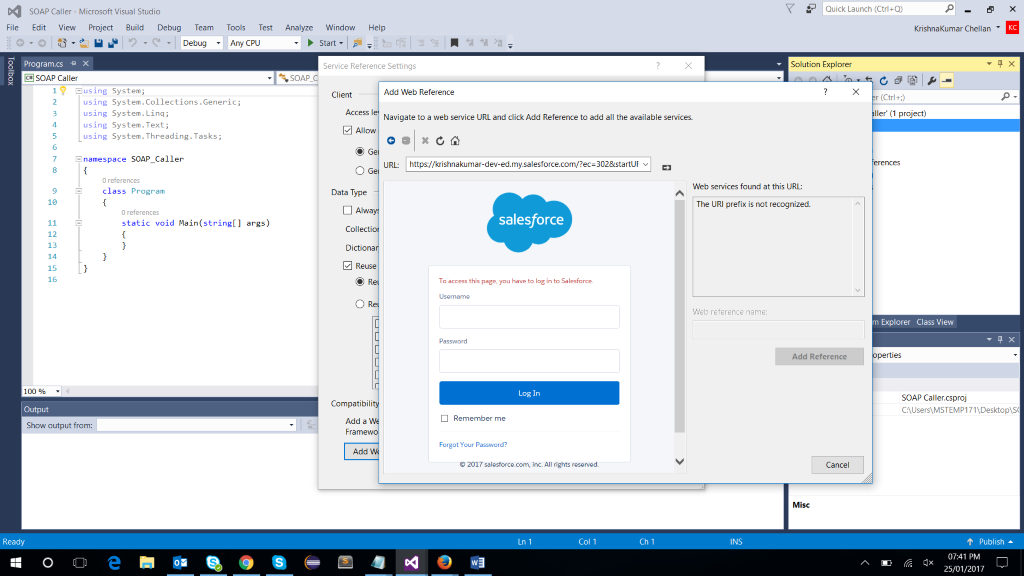
Step 7: After logging into Salesforce, it is automatically configured with Visual Studio.
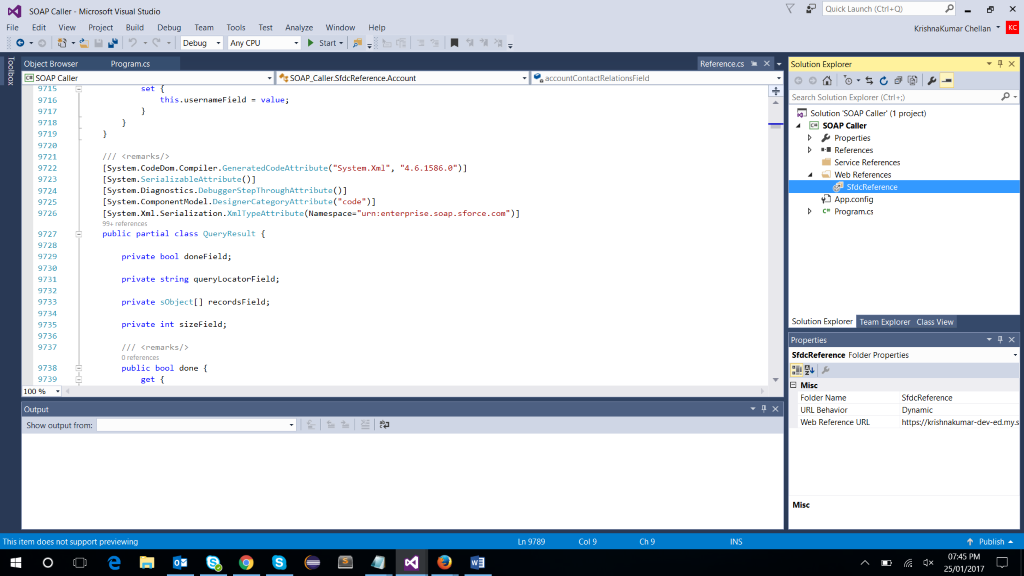
Establish API reference within Salesforce:Â
Here, the below source code deals with the different levels of operations on the Salesforce Contact object.
Source Code:
class Program { private static string sessionId = string.Empty; private static string serverUrl = string.Empty; static void Main(string[] args) { AuthenticateSfdcPartnerUser(); while (true) { Console.WriteLine("\n\t========== Menu ===========\n"); Console.WriteLine(" Press 1 for View Contact "); Console.WriteLine(" Press 2 for Add New Contact"); Console.WriteLine(" Press 3 for Search Contact "); Console.WriteLine(" Press 4 for Exit"); Console.WriteLine ("\n\t============================"); Console.Write("\nEnter your option:\t"); int opt = Convert.ToInt32(Console.ReadLine()); switch (opt) { case 1: Program.SelectRecord(); break; case 2: Program.CreateRecords(); break; case 3: Program.SearchRecord(); break; case 4: Environment.Exit(0); break; default: Console.WriteLine("Sorry, Invalid selection"); break; } } } private static void AuthenticateSfdcPartnerUser() { Console.WriteLine("Authenticating against the Partner API ..."); using (partner.SoapClient loginClient = new partner.SoapClient("Soap1")) { string sfdcPassword = "*********************"; string sfdcToken = "************************"; string sfdcUserName = "**********************"; string loginPassword = sfdcPassword + sfdcToken; partner.LoginResult result =loginClient.login (null,null,sfdcUserName, loginPassword); sessionId = result.sessionId; serverUrl = result.serverUrl; Console.WriteLine(""); Console.WriteLine("Press [Enter] to continue ..."); Console.ReadLine(); } } private static void SelectRecord() { try { EndpointAddress apiAddr = new EndpointAddress(serverUrl); partner.SessionHeader header = new partner.SessionHeader(); header.sessionId = sessionId; using (partner.SoapClient queryClient = new partner.SoapClient("Soap", apiAddr)) { string query = "SELECT Name,Company,Email FROM Lead"; partner.QueryResult result = queryClient.query(header, null, null, null, query); IEnumerable leadList = result.records.Cast(); Console.WriteLine("{0}{1}{2}", "Name".PadRight(30), "Company".PadRight(40), "Email".PadRight(60)); foreach (var leadItems in leadList) { Console.WriteLine("{0}{1}{2}", ((string)(leadItems.Name)).PadRight(30), ((string)(leadItems.Company)).PadRight(40), ((string)(leadItems.Email)).PadRight(60)); } Console.WriteLine(""); Console.WriteLine("Query complete."); Console.ReadLine(); } } catch (Exception) { throw; } } private static void CreateRecords() { EndpointAddress apiAddr = new EndpointAddress(serverUrl); partner.SessionHeader header = new partner.SessionHeader(); header.sessionId = sessionId; using (partner.SoapClient createClient = new partner.SoapClient("Soap", apiAddr)) { Console.Write("\nPlease Enter your Name : "); string name = Console.ReadLine(); Console.Write("\nPlease Enter your Company : "); string company = Console.ReadLine(); Console.Write("\nPlease Enter your Email : "); string email = Console.ReadLine(); Console.Write("\nPlease Enter your LeadSource : "); string leadSource = Console.ReadLine(); partner.Lead newLead = new partner.Lead(); newLead.LastName = name; newLead.Company = company; newLead.Email = email; newLead.LeadSource = leadSource; partner.SaveResult[] results; createClient.create(header, null, null, null, null, null, null, null, null, null, new partner.sObject[] { newLead }, out results); if (results[0].success) { Console.WriteLine("\n Lead successfully created."); } else { Console.WriteLine (results[0].errors[0]. message); } Console.ReadLine(); } } private static void SearchRecord() { EndpointAddress apiAddr = new EndpointAddress(serverUrl); partner.SessionHeader header = new partner.SessionHeader(); header.sessionId = sessionId; using (partner.SoapClient queryClient = new partner.SoapClient("Soap", apiAddr)) { Console.Write("\nPlease Enter your Id: "); string id = Console.ReadLine(); partner.sObject[] retrievedAccounts = queryClient.retrieve(header, null, null, null, "Name,Company,Email", "Lead", new string[] { id } ); Console.WriteLine("\n\n{0}{1}{2}", "Name".PadRight(30), "Company".PadRight(40), "Email".PadRight(60)); foreach (partner.sObject so in retrievedAccounts) { partner.Lead con = (partner.Lead)so; Console.WriteLine("\n{0}{1}{2}", ((string)(con.Name)).PadRight(30), ((string)(con.Company)).PadRight(40), ((string)(con.Email)).PadRight(60)); } Console.WriteLine(""); Console.WriteLine("Retrieve complete."); Console.ReadLine(); } } }
Step 8:Â Run the corresponding application in the console.
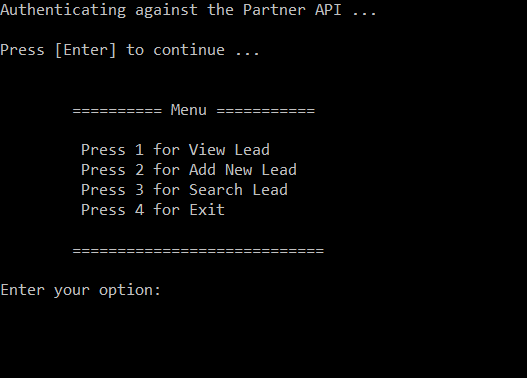
Step 9: Choose 1 and to view the existing contact records in salesforce.
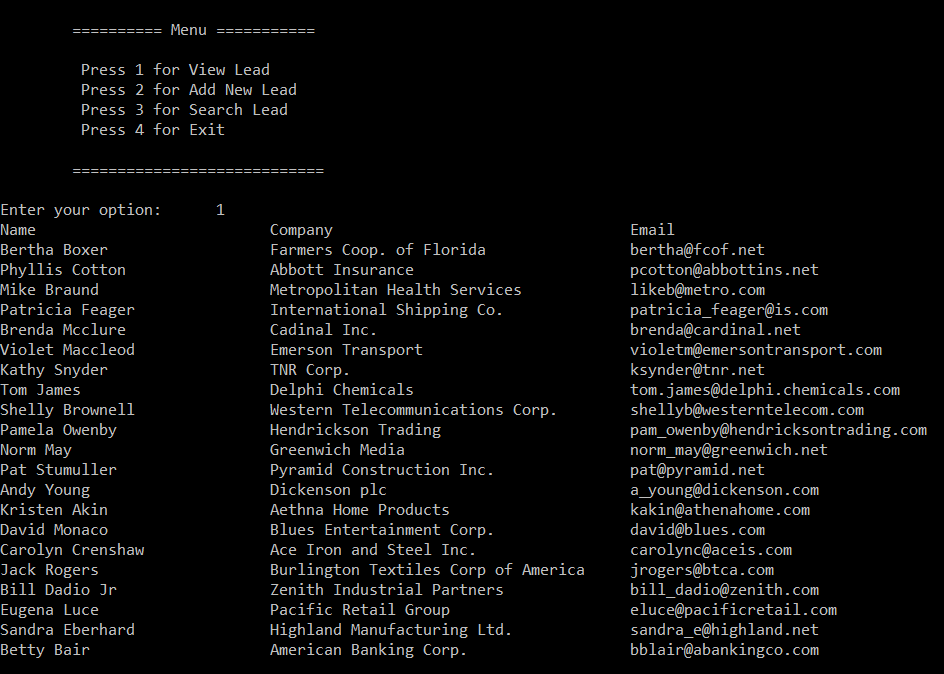
Step 10: Choose 2 for adding Contacts into the salesforce.
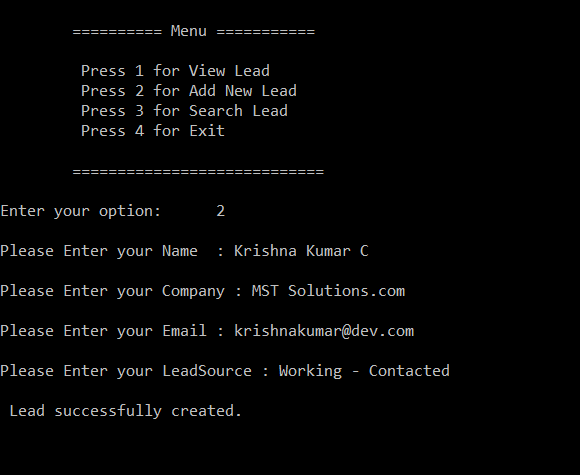
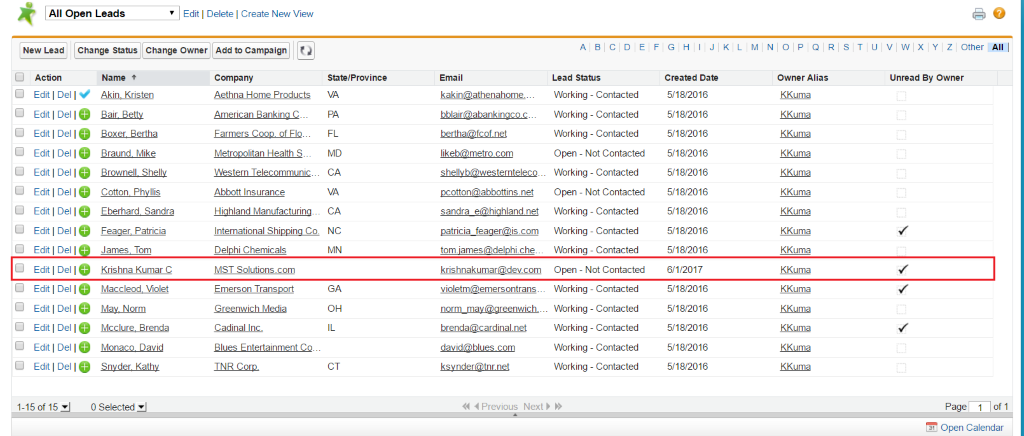
See the above contact record added in salesforce.
Step 11: Choose 3 to serach contacts from the Salesforce.
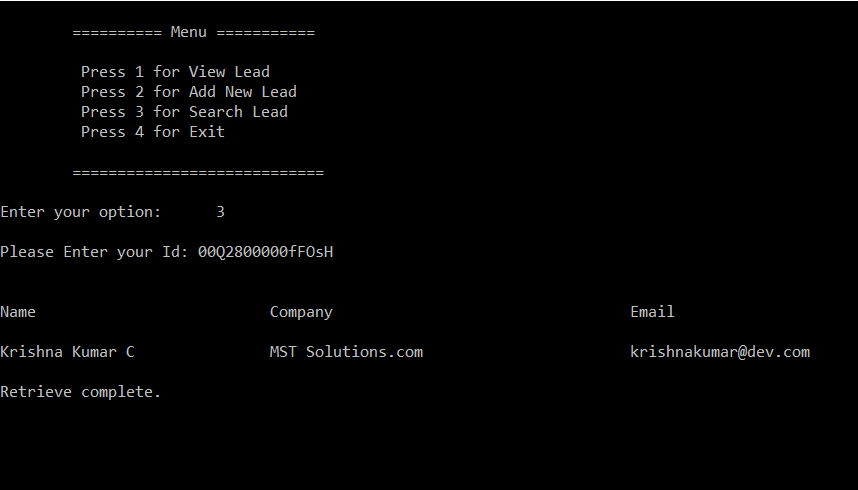
Step 12: Choose 4 to exit the current application.