Node.js
Node.js is an open source JavaScript framework. It is used to create single-page web applications, video streaming sites, and so on.
Node Package Manager (NPM)
NPM registry is an online repository for Node.js. The command line client used to install packages. It also does version management and dependency management.
Heroku Connect
Heroku Connect is an Add-on provided by Heroku. It helps us to connect web applications with Salesforce. Using Heroku connect we can automatically sync salesforce data with Heroku’s web application. It supports two-way synchronization. We can read and write data to Salesforce using Heroku.
Drawbacks
- Using Heroku Connect, we cannot conditionally synchronize salesforce data. It displays all the records for the connected SObjects. This can cause security threats.
- If we establish two-way synchronization, deletion of any record in the Heroku App also deletes the Salesforce record.
- The polling and synchronization of data is time consuming.
- Heroku Connect is expensive.
nForce
nForce is a package available in the npm registry. It acts a Salesforce REST API Wrapper for Salesforce.com, Force.com, and Database.com.
We can connect Node.js web application with Salesforce using nForce and manipulate Salesforce SObjects like Insert, Update, Delete and Query the sObjects using nForce.
We can retrieve records from Salesforce using SOQL query.
Prerequisite
- Create an Heroku account.
- Create an App in the Heroku account.
- Create the Front-End UI using HTML to view and manipulate the records.
Flow Diagram
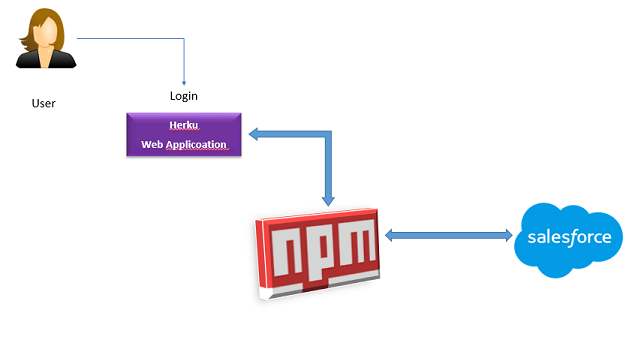
Installation
$ npm init - is used to interactively create the app’s package.json file. $ npm install - is used to install all packages defined in the package.json file required for the app. The following is the backend code which connects the Heroku app with Salesforce using Node.js. SERVER.JS var express = require("express"); var bodyParser = require("body-parser"); var nforce = require("nforce"); // Installing nforce module var app = express(); app.use(express.static(__dirname + "/public")); app.use(bodyParser.json()); //nforce setup to connect Salesforce var org = nforce.createConnection({ clientId: "ENTER THE SALESFORCE CONSUMER _KEY", clientSecret: "ENTER THE SALESFORCE CONSUMER_SECRECT", redirectUri: "https://APPNAME.herokuapp.com/oauth/_callback", apiVersion: "v37.0", // optional, defaults to current salesforce API version environment: "production", // optional, salesforce 'sandbox' or 'production', production default mode: "single" // optional, 'single' or 'multi' user mode, multi default }); // Initialize the app. var server = app.listen(process.env.PORT || 8080, function () { var port = server.address().port; console.log("App now running on port", port); }); // LEAD API ROUTES BELOW // Generic error handler used by all endpoints. function handleError(res, reason, message, code) { console.log("ERROR: " + reason); res.status(code || 500).json({"error": message}); } /* * GET: finds all Lead */ app.get("/lead", function(req, res) { org.authenticate({ username: 'SALESFORCE_USERNAME', password: 'PASSWORD_AND_SECURITY_TOKEN'}, function(err, oauth){ if(err) { console.log('Error: ' + err.message); } else { console.log('Access Token: ' + oauth.access_token); org.query({query:"select id, name, phone, website, createddate, lastmodifieddate from lead"}, function (err, resp) { if(err) throw err; if(resp.records && resp.records.length){ res.send(resp.records); } }); } }); });
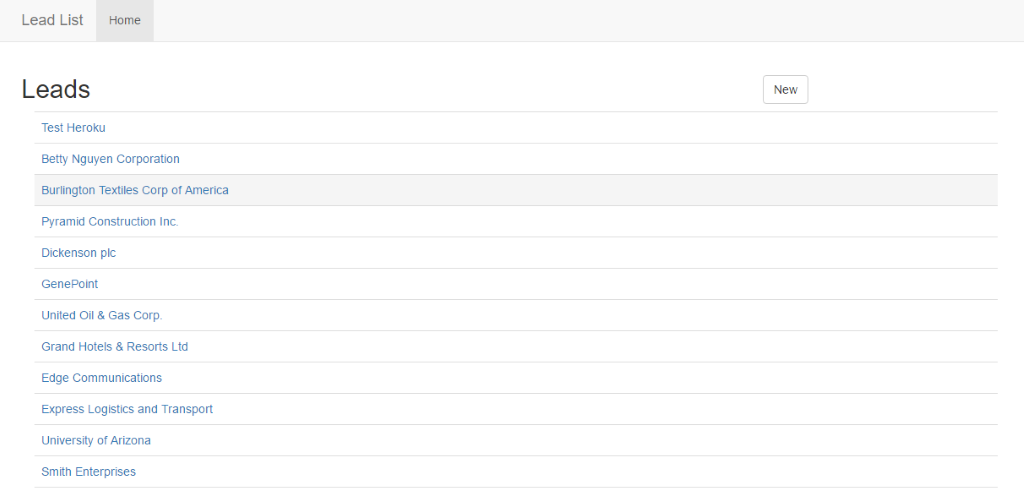
/* * POST: creates a new Lead */ app.post("/lead", function(req, res) { var newLead = req.body; if (!(req.body.name)) { handleError(res, "Invalid user input", "Please enter the Name.", 400); } console.log('Attempting to insert Lead'); var act = nforce.createSObject('Lead', newLead); org.insert({ sobject: act }, function(err, resp) { if(err) { console.error('--> unable to insert Lead'); console.error('--> ' + JSON.stringify(err)); } else { console.log('--> Lead inserted'); res.send(resp); } }); });
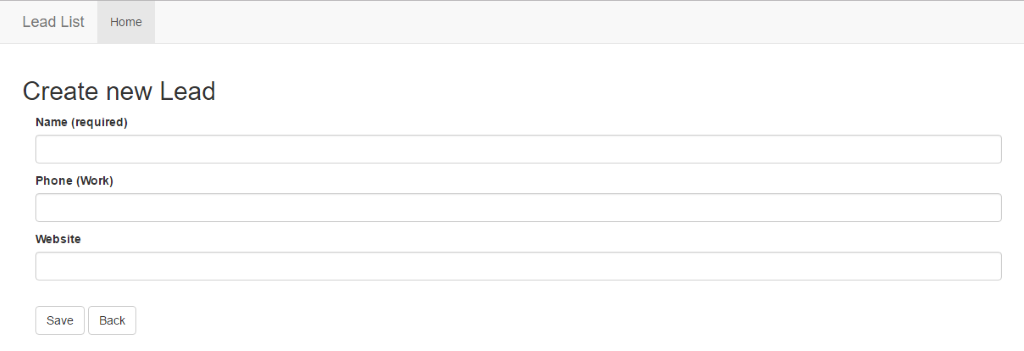
/* * GET: find Lead by id */ app.get("/lead/:id", function(req, res) { console.log('attempting to get the Lead'); org.getRecord({ type: 'lead', id: req.params.id }, function(err, act) { if(err) { console.error('--> unable to retrieve lead'); console.error('--> ' + JSON.stringify(err)); } else { console.log('--> Lead retrieved'); res.status(201).send(act); } }); });
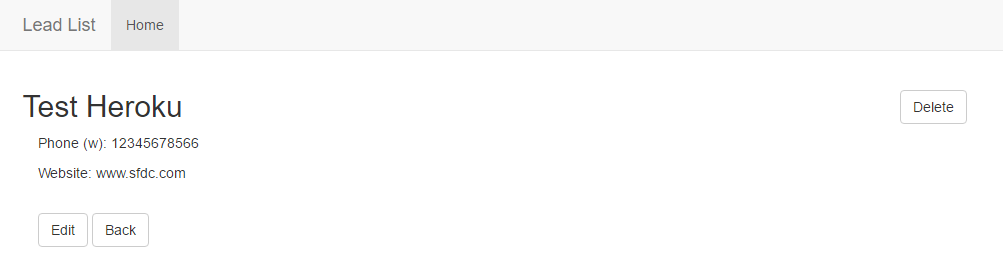
/* * PUT: update Lead by id */ app.put("/lead/:id", function(req, res) { var lead = req.body; if (!(req.body.name)) { handleError(res, "Invalid user input", "Please enter the Name.", 400); } console.log('Attempting to update Lead'); var act = nforce.createSObject('Lead', { id : req.params.id, name : lead.name, phone : lead.phone }); org.update({ sobject: act }, function(err, resp) { if(err) { console.error('--> unable to update Lead'); console.error('--> ' + JSON.stringify(err)); } else { console.log('--> Lead updated'); res.status(204).end(); } }); });
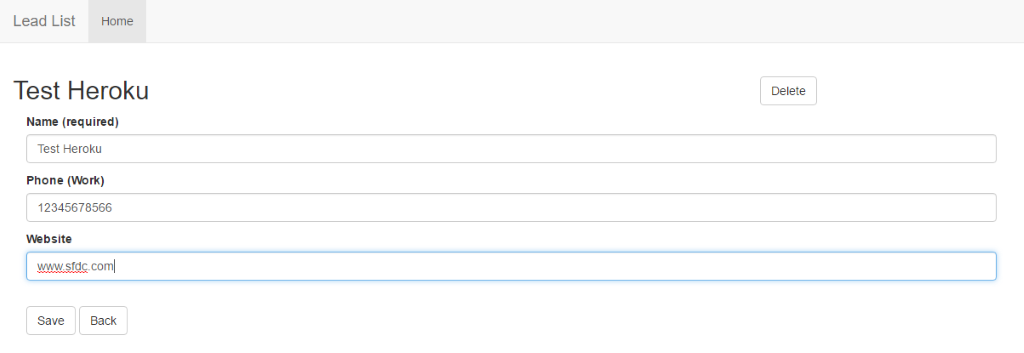
/* * DELETE: deletes Lead by id */ app.delete("/lead/:id", function(req, res) { var leadId = req.params.id; console.log('this is' + req.params.id); var act = nforce.createSObject('lead', { id : leadId }); org.delete({sobject : act}, function(err, act) { if(err) { console.error('--> unable to retrieve lead'); console.error('--> ' + JSON.stringify(err)); } else { console.log('--> Lead deleted'); res.status(204).end(); } }); });
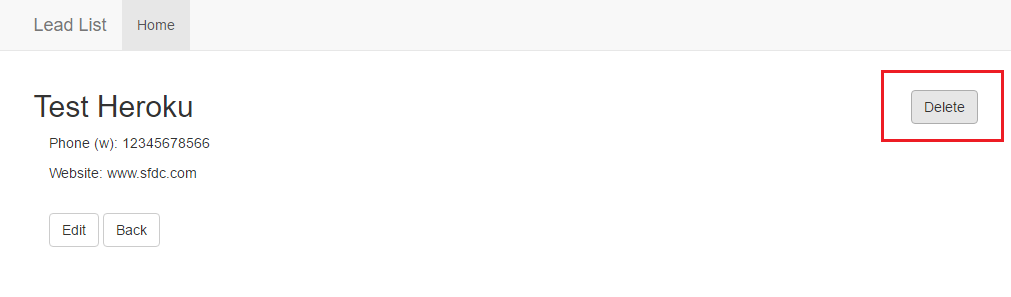