In DocuSign, we can update the recipient once the envelop has been sent. When you update the recipient, the envelop must be in “In Process” status. You cannot update the recipient when the envelop is “Completed” or “Void” status.
For this you must have the following things.
- You must have a DocuSign account.
- Install DocuSign app in Salesforce account.
- Enable the API key into your DocuSign account.
- Store the DocuSign credential and API key into your custom setting in salesforce.
For updating the recipient into the envelop, you need to send the callout to the following endpoint
/accounts/{accountId}/envelopes/{envelopeId}
/recipients
For sending callout to the above endpoint we should have envelopId, so we can store the envelop id in one hidden field. For my scenario, I have stored the envelopId in Envelop_Id__c field in case object.
Here is the code snippet for updating the recipient in the Envelop. You can execute this snippet in your developer console
String account; String UserName; String Password; String IntegratorKey; String endPointURL; String templateID; String endpoint; String json; String aaa = '?advanced_update=true'; Map<String,DocuSignCredentialsSetting__c> credentialSetting = DocuSignCredentialsSetting__c.getAll(); case currentParentCase = [SELECT Id,CaseNumber,Envelop_Id__c FROM Case WHERE Id =: '5000n000000pTjU']; //Get the docusign credential details from the custom setting. for(DocuSignCredentialsSetting__c credentialIns : credentialSetting.values()){ UserName = credentialIns.name; account = credentialIns.account__c; Password = credentialIns.Password__c; IntegratorKey = credentialIns.IntegratorKey__c; endPointURL = credentialIns.end_Point__c; } //docusign template Id templateID = label.WishClearence; if(currentParentCase.Envelop_Id__c == Null) endpoint = endPointURL+'/accounts/'+account+' /envelopes'; else endpoint = endPointURL+'/accounts/'+account+' /envelopes/'+currentParentCase. Envelop_Id__c+'/recipients'; system.debug('### endpoint #####'+endpoint ); String authorizationHeader = '<DocuSignCredentials><Username>'+UserName+'</Username><Password>'+Password+'</Password><IntegratorKey>'+IntegratorKey+' </IntegratorKey></DocuSignCredentials>'; HttpRequest req = new HttpRequest(); req.setEndpoint(endPoint); if(currentParentCase.Envelop_Id__c == Null) req.setMethod('POST'); else req.setMethod('PUT'); req.setHeader('X-DocuSign-Authentication', authorizationHeader); req.setHeader('Accept','application /json'); req.setHeader('Content-Length','162100'); req.setHeader('Content-Type','application /json'); req.setHeader('Content-Disposition','form-data'); if(currentParentCase.Envelop_Id__c == Null) { json='{'+ ' '+ ' "emailSubject": "This is email subject",'+ ' "emailBlurb": "This is emailblurb",'+ ' "templateId": "'+templateID+'",'+ ' "envelopeIdStamping": "false",'+ ' "customFields":{'+ ' "textCustomFields":['+ ' {'+ ' "name":"##SFCase",'+ ' "required":"true",'+ ' "show":"false",'+ ' "value":"'+currentParentCase.Id+'"'+ ' }'+ ' ]'+ ' },'+ ' "templateRoles": ['+ '{'+ '"roleName": "Signer 1",'+ '"name": "'+'kanagaraj'+'",'+ '"email": "'+'kanagaraj@mstsolutions.com'+'",'+ '"emailNotification": {'+ '"emailSubject": "Signature Required-Wish Clearance ",'+ '},'+ '},'+ ' ],'+ ' "status": "sent"'+ '}'; } else{ json='{'+ ' '+ ' "signers": ['+ '{'+ '"roleName": "Signer 1",'+ '"recipientId":"1",'+ '"name": "'+'Vijy'+'",'+ '"email": "'+'Vijay@mstsolutions.com'+'",'+ '"emailNotification": {'+ '"emailSubject": "Signature Required-Wish Clearance ",'+ '},'+ '},'+ ' ],'+ ' "status": "sent"'+ '}'; } req.setBody(json); Http http = new Http(); HTTPResponse res; try{ res = http.send(req); system.debug('DocuSign Response'+ res.getBody()); } catch(Exception e){ ApexPages.addMessage( new ApexPages.Message(ApexPages.Severity. FATAL, e.getMessage())); } WrapperDocuSign wrapInst = WrapperDocuSign.parse(res.getBody()); IF(currentParentCase.Envelop_Id__c == Null) { currentParentCase.Envelop_Id__c = wrapInst.envelopeId; Update currentParentCase; } system.debug('WrapperDocusign'+wrapInst); system.debug('Current Case Id'+currentParentCase.Id); // This wrapper class is used to parse the Jason response that is received from the docusign public class WrapperDocuSign { public String envelopeId; public String uri; public String statusDateTime; public String status; public static WrapperDocuSign parse(String json){ return (wrapperDocusign)System.JSON. deserialize(JSON,WrapperDocuSign.class); } }
Result :
Here, we are making a callout to DocuSign with the recipient as “Kanagaraj”
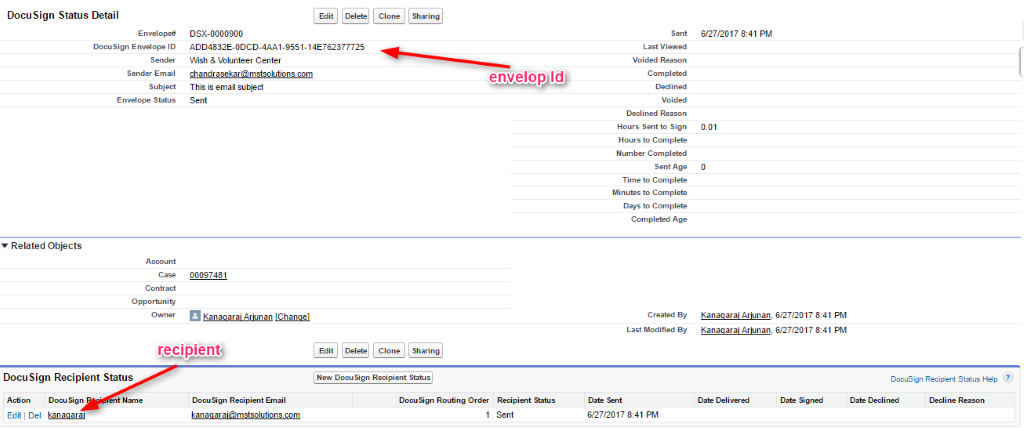
Here, we are making a callout to update the recipient with the name as “Vijay”
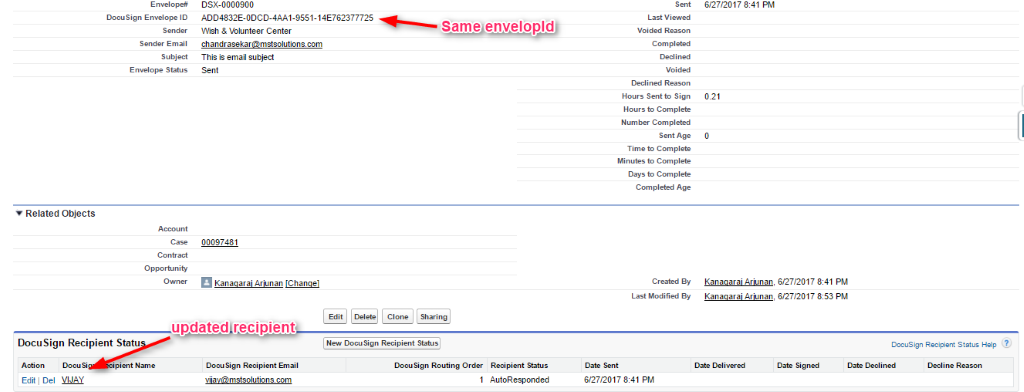
Reference Link
https://www.docusign.com/p/RESTAPIGuide/
Content/REST%20API%20References/add-or-modify-envelope-information.htm